In your quest to tackle Python interviews, ‘Top 500+ Python Interview Questions’ serves as your comprehensive guide. Covering Python Basics, Data Structures, Functions and Modules, Object-Oriented Programming, and Advanced Python Concepts, this resource equips you with the knowledge needed to ace those interviews.
With a vast array of questions at your disposal, you can sharpen your Python skills and boost your confidence when facing potential employers. Dive into this collection to enhance your understanding and prepare yourself for a successful interview journey.
Python Basics
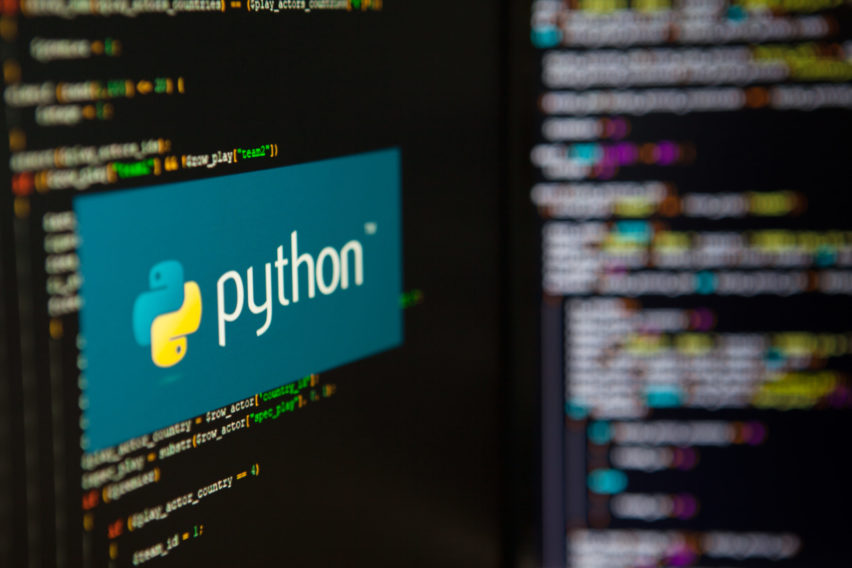
- What is Python and why is it called “Python”?
- Differentiate between Python 2 and Python 3.
- Explain the term “Pythonic.”
- What are the key features of Python?
- Discuss the Zen of Python.
- How is memory managed in Python?
- Explain the Global Interpreter Lock (GIL).
- What is the purpose of the
sys.argv
list? - Differentiate between local and global variables in Python.
- Explain the use of the
pass
statement in Python.
Data Types and Variables
- What are the basic data types in Python?
- Explain the concept of mutability.
- How does string interpolation work in Python?
- Describe the purpose of the
is
operator. - What is a docstring, and how is it used?
- Discuss the
id()
function in Python. - Explain the use of the
format()
method for string formatting. - How are lists and tuples different in Python?
- What is dictionary comprehension?
- Discuss the purpose of the
None
value in Python.
Control Flow
- Explain the purpose of the
if-elif-else
statement. - How is the
switch
statement emulated in Python? - Describe the role of the
range()
function. - Explain the use of list comprehensions.
- Discuss the purpose of the
enumerate()
function. - How does exception handling work in Python?
- Explain the use of the
with
statement. - Discuss the differences between
continue
andbreak
. - What is the purpose of the
assert
statement? - How are iterators and generators different?
Functions
- How are arguments passed in Python – by reference or value?
- Explain the concepts of first-class functions and higher-order functions.
- Discuss the differences between
*args
and**kwargs
. - What is a closure in Python?
- Explain the concept of decorators.
- Discuss the use of lambda functions.
- How is recursion implemented in Python?
- Explain the difference between
return
andyield
in functions. - What is a docstring, and why is it important?
- How do you handle default values for function parameters?
Object-Oriented Programming (OOP)
- Define class and object in Python.
- Explain the concepts of encapsulation, inheritance, and polymorphism.
- Discuss the differences between class and instance variables.
- What is the purpose of the
super()
function? - How is method overloading achieved in Python?
- What is the purpose of the
__init__
method? - Explain the role of
self
in Python classes. - Discuss the use of class methods and static methods.
- How are abstract classes implemented in Python?
- Explain multiple inheritance in Python.
Exception Handling
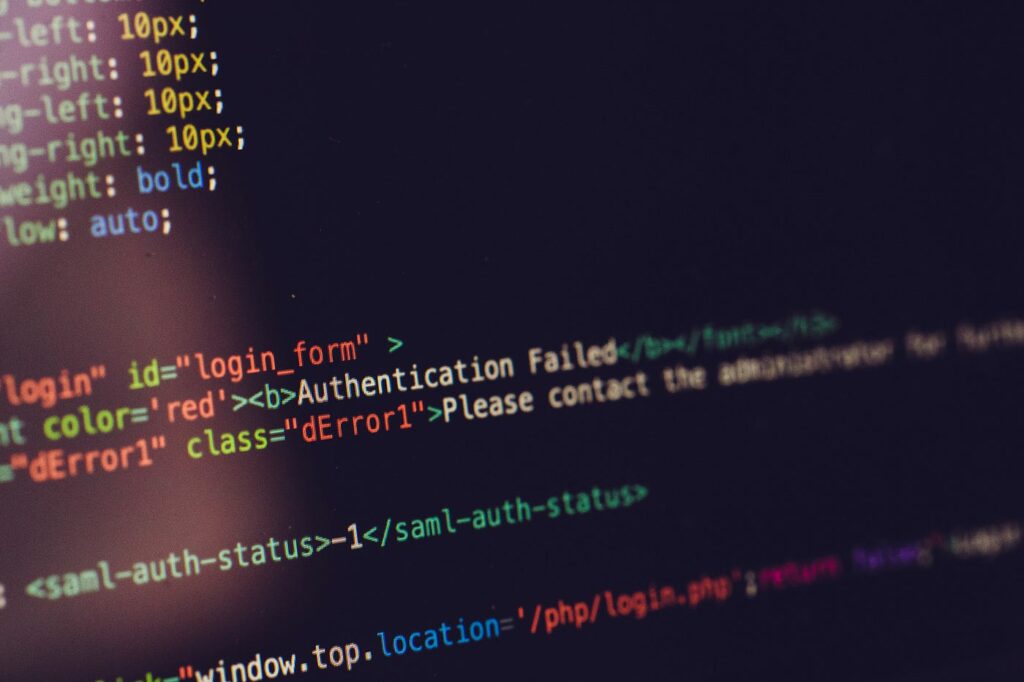
- How is exception handling implemented in Python?
- Discuss the differences between
try
,except
, andfinally
blocks. - What is the purpose of the
else
clause in exception handling? - Explain the concept of custom exceptions.
- How can you use the
assert
statement for debugging?
File Handling
- How do you open and close a file in Python?
- Discuss the differences between reading modes (
r
,rb
,r+
) in file handling. - What is the purpose of the
with
statement in file handling? - Explain the use of the
seek()
method. - How can you read and write CSV files in Python?
Modules and Packages
- What is a module in Python?
- Explain the purpose of the
__init__.py
file. - How do you import modules in Python?
- Discuss the differences between
import module
andfrom module import *
. - What is a package, and how is it different from a module?
Regular Expressions
- What are regular expressions, and how are they used in Python?
- Discuss the functions provided by the
re
module. - How can you match multiple occurrences in a regular expression?
- Explain the use of character classes in regular expressions.
- How is the
sub()
function used for substitution?
Advanced Python Concepts
- Discuss the importance of the Global Interpreter Lock (GIL).
- Explain the purpose of the
__name__
variable. - How are context managers implemented in Python?
- What are metaclasses, and how are they used?
- Discuss the role of the
__future__
module. - Explain the purpose of the
sys
module. - What is the purpose of the
__slots__
attribute? - How does garbage collection work in Python?
- Discuss the differences between CPython, Jython, and IronPython.
- Explain the purpose of the
itertools
module.
Libraries and Frameworks
- Discuss the significance of NumPy in scientific computing.
- What is the purpose of the pandas library?
- Explain the role of Flask in web development.
- Discuss the components of the Django web framework.
- How is asynchronous programming achieved in Python using asyncio?
- What is the purpose of the
requests
library? - Discuss the importance of the
BeautifulSoup
library in web scraping. - Explain the role of the
scikit-learn
library in machine learning. - How does TensorFlow contribute to deep learning in Python?
- What are the advantages of using PyTorch for neural network development?
Web Development with Python
- Discuss the concept of WSGI in Python web development.
- What is the purpose of middleware in a web application?
- Explain the role of a web server in Python web development.
- How does the Model-View-Controller (MVC) pattern apply to web development in Python?
- Discuss the significance of ORM (Object-Relational Mapping) in web development.
Flask Framework
- How does routing work in the Flask web framework?
- What are Flask blueprints, and how are they used?
- Explain the purpose of Flask templates.
- How can you handle form data in Flask?
- Discuss the use of Flask extensions in web development.
Django Framework
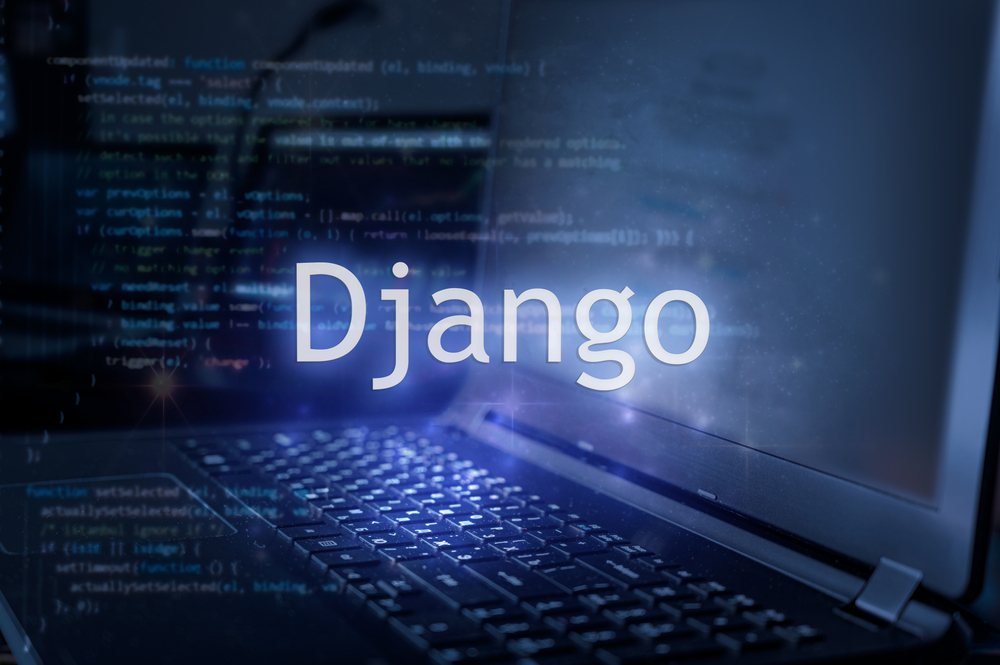
- What is Django, and how does it differ from other web frameworks?
- How is Django’s URL routing system configured?
- Explain the purpose of Django models.
- How does Django handle database migrations?
- Discuss Django’s template system.
- What are Django signals, and how are they used?
- Explain the concept of Django middleware.
- How does Django manage user authentication and authorization?
Testing in Python
- Describe the purpose of the
unittest
module. - How can you write unit tests for Python code?
- Discuss the use of mocking in Python testing.
- What is test-driven development (TDD), and how can it be applied in Python?
- Explain the concept of fixtures in testing.
Database Connectivity
- How do you connect to a database in Python?
- Discuss the differences between SQLite and MySQL.
- What is an ORM (Object-Relational Mapping), and how is it used in Python?
- Explain the purpose of database connection pooling.
- How can you execute raw SQL queries in Django?
Networking in Python
- Explain the purpose of the
socket
module in Python. - How does HTTP communication work in Python?
- Discuss the use of the
requests
library for HTTP requests. - What is a RESTful API, and how is it implemented in Python?
- How can you perform asynchronous networking in Python using asyncio?
Multithreading and Multiprocessing
- Discuss the differences between multithreading and multiprocessing.
- Explain the Global Interpreter Lock (GIL) and its impact on multithreading.
- How can you achieve parallelism in Python using the
concurrent.futures
module? - Discuss the use of the
threading
andmultiprocessing
modules.
Python Packaging:
- What is a virtual environment, and why is it used?
- Explain the purpose of the
requirements.txt
file. - How can you create a Python package for distribution?
- Discuss the differences between
setup.py
andpyproject.toml
for package configuration. - Explain the purpose of the
__init__.py
file in a Python package.
Python Best Practices
- Describe PEP 8 and its significance in Python coding standards.
- What is the purpose of docstrings, and how should they be formatted?
- Discuss the importance of version control in Python projects.
- How can you manage dependencies in a Python project?
- Explain the use of type hints in Python, and how they improve code quality.
- Discuss the importance of testing and continuous integration in Python development.
- How can you optimize Python code for better performance?
- What are virtual environments, and why are they recommended for Python development?
Data Science and Machine Learning with Python
- Explain the role of Python in data science.
- Discuss the significance of NumPy and Pandas in data analysis.
- How can you visualize data in Python using Matplotlib and Seaborn?
- Discuss the use of Jupyter Notebooks in data science.
- Explain the purpose of the
scikit-learn
library in machine learning. - What is the difference between supervised and unsupervised learning?
- How can you evaluate the performance of a machine-learning model in Python?
- Discuss the role of neural networks and deep learning in Python.
- How does TensorFlow contribute to deep learning in Python?
- Explain the purpose of the PyTorch library in machine learning.
Web Scraping with Python
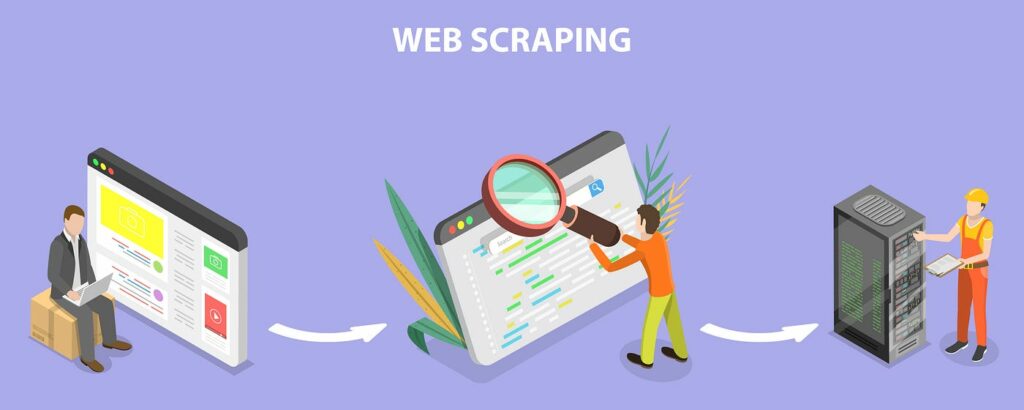
- Describe the steps involved in web scraping with Python.
- How can you use the
requests
library for web scraping? - Explain the purpose of the
BeautifulSoup
library in parsing HTML. - Discuss the differences between web scraping and web crawling.
- How do you handle dynamic content in web scraping?
- Explain the use of Selenium for web scraping in Python.
- How can you avoid being blocked while web scraping?
- Discuss ethical considerations and legal aspects of web scraping in Python.
Python and Automation
- How can Python be used for automation tasks?
- Explain the use of the
os
module in file and directory operations. - Discuss the significance of the
shutil
module in Python automation. - How can you schedule tasks in Python using
cron
orschedule
? - What is the purpose of the
subprocess
module in Python? - Explain the use of the
logging
module for generating logs in Python. - How can you automate web interactions using Selenium in Python?
GUI Programming with Python
- Discuss the options for GUI programming in Python.
- What is Tkinter, and how is it used for GUI development?
- Explain the purpose of PyQT in Python GUI programming.
- Discuss the use of Kivy for developing cross-platform GUI applications.
- How can you create graphical interfaces using wxPython?
Security in Python
- Discuss common security vulnerabilities in Python applications.
- Explain the purpose of the
hashlib
module for secure hashing. - How can you handle user authentication securely in a Python web application?
- Discuss best practices for securing RESTful APIs in Python.
- How can you implement secure file uploads in a Python application?
Docker and Python
- How can Docker be used with Python applications?
- Discuss the advantages of containerization in Python projects.
- Explain the purpose of a Dockerfile in Python Dockerization.
- How can you orchestrate multiple containers using Docker Compose?
- Discuss challenges and best practices for deploying Python applications with Docker.
Deployment and Scaling
- Describe common methods for deploying Python applications.
- How can you use platforms like Heroku for Python application deployment?
- Discuss the advantages of deploying Python applications on cloud services like AWS or Azure.
- How can you scale a Python application to handle increased traffic?
- Discuss the use of load balancing for scaling Python applications.
Python Interview Questions – Algorithmic and Problem Solving
- Implement a function to check if a string is a palindrome.
- Write a program to find the factorial of a number.
- Implement a binary search algorithm in Python.
- Solve the classic “FizzBuzz” problem.
- Write a function to find the largest element in a list.
- Implement a stack and perform basic operations on it.
- Write a program to reverse a linked list.
- Implement a queue using two stacks.
- Write a function to check if two strings are anagrams.
- Implement a depth-first search (DFS) algorithm on a graph.
- Write a program to solve the Tower of Hanoi problem.
- Implement a basic calculator for arithmetic expressions.
- Write a function to find the shortest path in a maze.
- Implement a bubble sort algorithm.
- Write a program to check if a given number is prime.
Data Structures
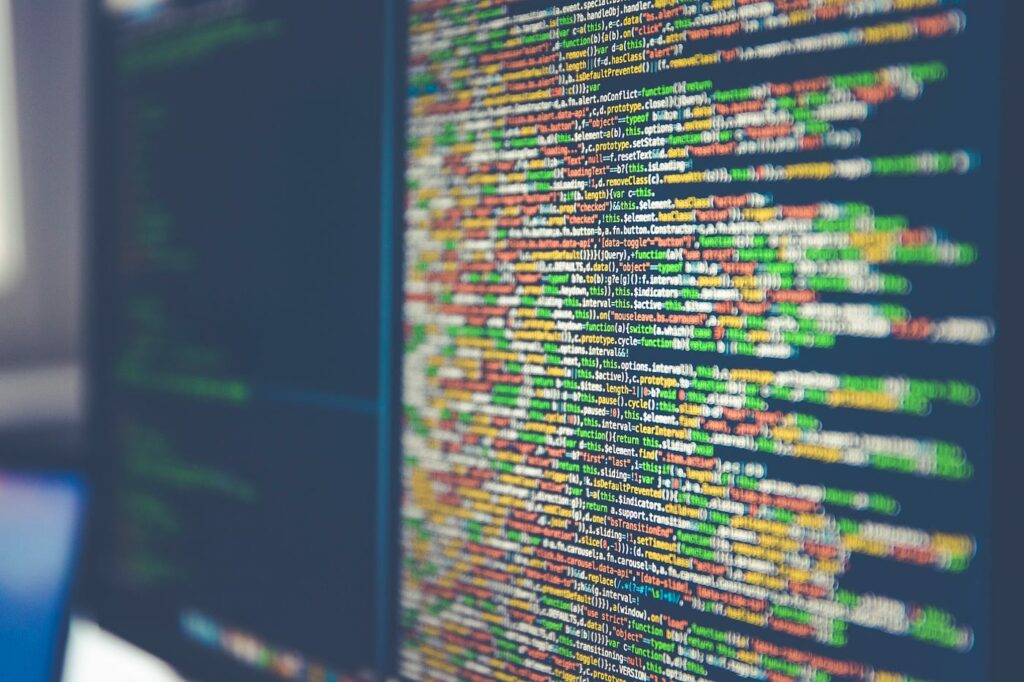
- Discuss the differences between arrays and linked lists.
- Explain the concept of a hash table and its implementation.
- How are trees and graphs different in data structures?
- Implement a binary tree in Python.
- What is a heap, and how is it used in Python?
- Discuss the advantages and disadvantages of various tree structures.
- How can you implement a priority queue in Python?
- Explain the differences between a stack and a queue.
- Implement a doubly linked list in Python.
- How is a trie used in Python?
Sorting and Searching
- Implement the quicksort algorithm in Python.
- Discuss the time complexity of various sorting algorithms.
- How does the binary search algorithm work?
- Write a program to perform a linear search in Python.
- Implement the merge sort algorithm in Python.
- Discuss the differences between stable and unstable sorting algorithms.
- How can you find the kth smallest element in an unsorted array?
- Implement the insertion sort algorithm in Python.
- Write a program to find the intersection of two sorted arrays.
- Discuss the concept of searching in a rotated sorted array.
Dynamic Programming
- Explain the concept of dynamic programming.
- Solve the Fibonacci sequence using dynamic programming.
- Write a program to find the longest common subsequence in two strings.
- Implement the knapsack problem using dynamic programming.
- Discuss the use of memoization in dynamic programming.
- Solve the coin change problem using dynamic programming.
- Write a program to find the longest increasing subsequence in an array.
- Implement the longest palindromic subsequence algorithm.
- Discuss the differences between top-down and bottom-up dynamic programming.
- Solve the rod-cutting problem using dynamic programming.
Graph Algorithms
- Discuss the differences between a directed and an undirected graph.
- Explain the concepts of breadth-first search (BFS) and depth-first search (DFS).
- Implement Dijkstra’s algorithm for finding the shortest path in a graph.
- Discuss the importance of connected components in a graph.
- How does Kruskal’s algorithm work for finding the minimum spanning tree?
- Explain the concepts of in-degree and out-degree in a directed graph.
- Discuss the differences between a tree and a forest in graph theory.
- How can you detect a cycle in a directed graph?
- Write a program to perform topological sorting in a directed acyclic graph (DAG).
- Discuss the applications of graph algorithms in real-world problems.
Tree Data Structures
- Implement a binary search tree (BST) in Python.
- Discuss the differences between a binary tree and a binary search tree.
- Explain the concepts of balanced and unbalanced trees.
- How does an AVL tree maintain balance?
- Implement a trie (prefix tree) in Python.
- Discuss the applications of trees in computer science.
- Explain the differences between a full binary tree and a complete binary tree.
- Write a program to find the lowest common ancestor in a binary tree.
- Discuss the concept of threaded binary trees.
- How can you serialize and deserialize a binary tree?
Database Design
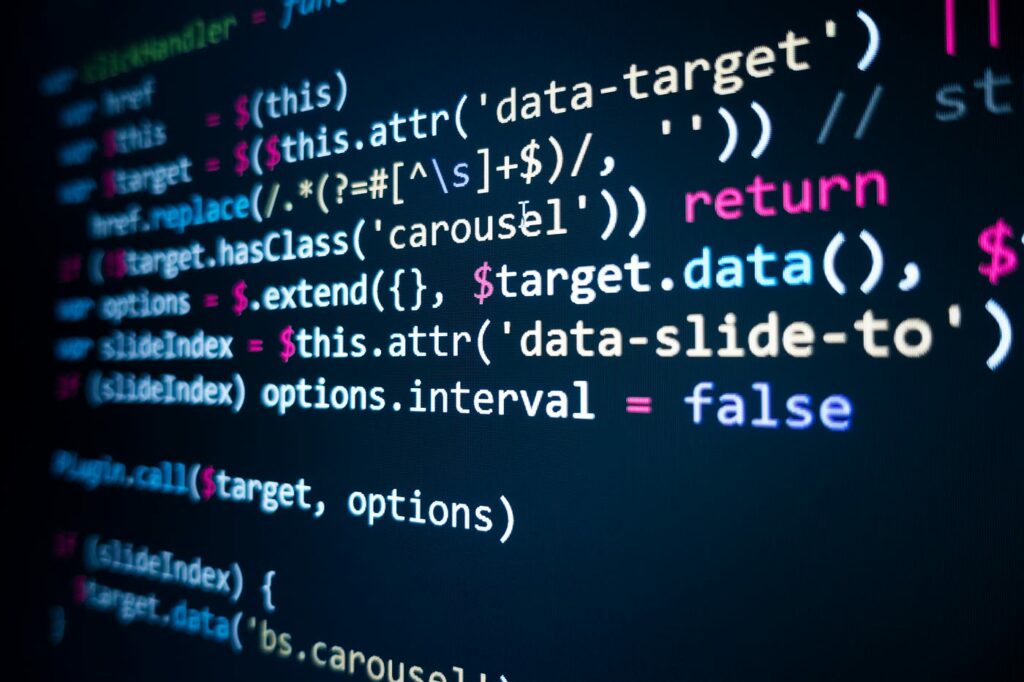
- Discuss the importance of database normalization.
- Explain the differences between the primary key and the foreign key.
- How can you optimize database performance in Python applications?
- Discuss the concept of denormalization and its use cases.
- Explain the purpose of indexing in a database.
- How can you model a many-to-many relationship in a relational database?
- Discuss the advantages and disadvantages of NoSQL databases.
- How is ACID (Atomicity, Consistency, Isolation, Durability) maintained in database transactions?
- Write a SQL query to find the second-highest salary in a table.
- Discuss the considerations for choosing a database for a Python application.
System Design
- Explain the importance of system design in software development.
- Discuss the differences between monolithic and microservices architectures.
- How can you design a scalable system architecture for a web application?
- Discuss the role of load balancing in system design.
- What is a Content Delivery Network (CDN), and how does it improve system performance?
- How can you design a fault-tolerant system architecture?
- Discuss the considerations for designing a database schema.
- Explain the concept of sharding in distributed databases.
- How can you design an authentication and authorization system for a web application?
- Discuss the importance of caching in system design.
Cloud Computing with Python
- Explain the role of Python in cloud computing.
- How can you deploy a Python application on a cloud platform like AWS or Azure?
- Discuss the advantages of serverless computing for Python applications.
- What is the purpose of an Infrastructure as a Service (IaaS) platform?
- How can you use cloud storage services like Amazon S3 in Python?
- Discuss the considerations for choosing a cloud provider for Python applications.
- How does Platform as a Service (PaaS) simplify Python application deployment?
- Explain the concept of auto-scaling in cloud computing.
- Discuss the challenges and solutions for data security in the cloud.
- How can you monitor and troubleshoot Python applications in the cloud?
RESTful APIs
- What is a RESTful API, and how is it implemented in Python?
- Discuss the principles of Representational State Transfer (REST).
- How can you handle authentication in a RESTful API?
- Explain the use of HTTP methods (GET, POST, PUT, DELETE) in RESTful APIs.
- Discuss the purpose of status codes in HTTP responses.
- How can you handle pagination in a RESTful API?
- What is the role of content negotiation in RESTful API design?
- Explain the concept of versioning in RESTful APIs.
- How can you implement rate limiting in a RESTful API?
- Discuss the use of HATEOAS (Hypermedia as the Engine of Application State) in RESTful APIs.
Machine Learning Algorithms
- Explain the concepts of supervised and unsupervised learning.
- What is the purpose of regression analysis in machine learning?
- Discuss the differences between classification and clustering algorithms.
- How can you evaluate the performance of a machine-learning model?
- Explain the concepts of precision and recall in machine learning.
- Discuss the role of feature engineering in machine learning.
- How does the k-nearest neighbours (KNN) algorithm work?
- Explain the purpose of decision trees in machine learning.
- Discuss the advantages and disadvantages of support vector machines (SVM).
- What is the purpose of ensemble learning in machine learning?
Natural Language Processing (NLP)
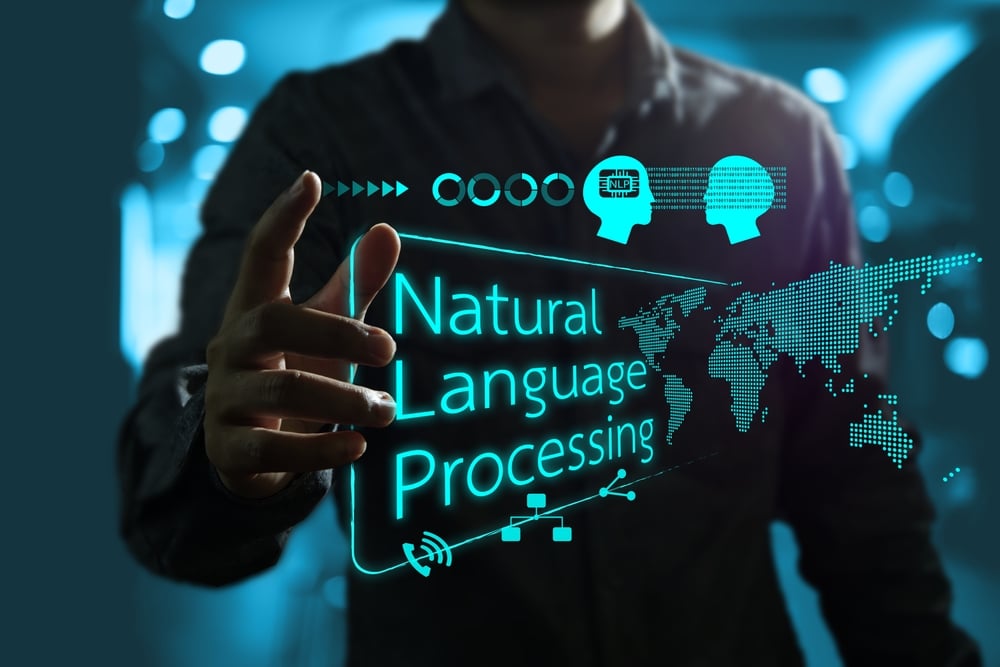
- How can Python be used in natural language processing (NLP)?
- Discuss the challenges of tokenization in NLP.
- How can you perform part-of-speech tagging in Python?
- What is named entity recognition (NER), and how is it implemented in Python?
- Explain the concept of stemming and lemmatization in NLP.
- How can you use machine learning for sentiment analysis in Python?
- Discuss the importance of feature extraction in NLP.
- What is topic modelling, and how can it be applied in NLP?
- How can you implement a chatbot using Python and NLP?
- Discuss the ethical considerations in NLP and language models.
Tensorflow and PyTorch
- Discuss the differences between TensorFlow and PyTorch.
- How can you create and train a neural network using TensorFlow?
- Explain the purpose of tensors in TensorFlow.
- What is transfer learning, and how is it implemented in TensorFlow?
- Discuss the role of Keras as a high-level API for deep learning.
- How can you use TensorFlow for natural language processing tasks?
- Explain the basics of building and training neural networks with PyTorch.
- Discuss the advantages of dynamic computation graphs in PyTorch.
- How can you save and load trained models in TensorFlow and PyTorch?
- Discuss the challenges and solutions for deploying deep learning models in production.
Python Concurrency
- Explain the Global Interpreter Lock (GIL) and its impact on Python concurrency.
- Discuss the differences between multithreading and multiprocessing.
- How can you achieve parallelism in Python using the
concurrent.futures
module? - What is asynchronous programming, and how is it implemented in Python?
- Discuss the use of the
asyncio
module for asynchronous programming. - How can you use the
threading
module for multithreading in Python? - Discuss the challenges of concurrency in Python and how they can be addressed.
- What is the purpose of the
queue
module in Python concurrency? - How can you use locks and semaphores to synchronize threads in Python?
- Discuss the advantages and disadvantages of using multiprocessing in Python.
Miscellaneous
- What is the purpose of the
__init__
a method in Python classes? - Discuss the use of the
zip()
function in Python. - How do you handle multiple exceptions in a single
except
block? - Explain the purpose of the
@staticmethod
decorator. - How can you iterate over multiple lists simultaneously in Python?
- Discuss the differences between shallow copy and deep copy in Python.
- What is the purpose of the
sys
module in Python? - How can you profile and optimize Python code for better performance?
- Discuss the use of the
itertools
module in Python. - How can you implement a singleton pattern in Python?
Python Interview Coding Challenges
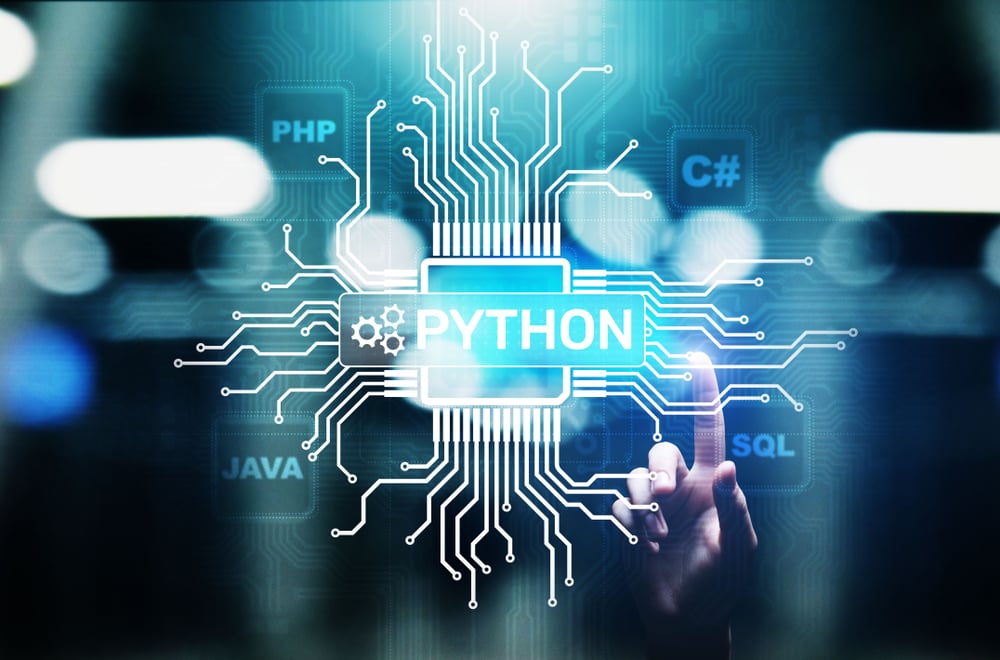
- Implement a function to reverse a string in Python.
- Write a program to check if a number is prime.
- Implement a queue using stacks in Python.
- Write a function to find the intersection of two lists in Python.
- Solve the “Two Sum” problem using Python.
- Implement a binary tree and perform depth-first and breadth-first traversals.
- Write a program to detect a cycle in a directed graph in Python.
- Implement a simple LRU (Least Recently Used) cache in Python.
- Solve the “Longest Common Subsequence” problem using dynamic programming.
- Write a program to find the shortest path in a maze using BFS.
- Implement a basic calculator for arithmetic expressions in Python.
- Solve the “Knapsack Problem” using dynamic programming in Python.
- Implement the quicksort algorithm in Python.
- Write a program to find the kth smallest element in an unsorted array.
- Implement a stack that supports push, pop, top, and retrieving the minimum element.
- Write a function to check if a binary tree is balanced.
- Implement an algorithm to check if a Sudoku board is valid.
- Solve the “Longest Increasing Subsequence” problem using dynamic programming.
- Implement the merge sort algorithm in Python.
- Write a program to perform a binary search on a sorted array.
… and many more!
See Also: Helpful Tips for Ensuring Profitable Product Launches
Keep in mind that Phython interview questions can vary based on the specific role and company. It’s essential to thoroughly understand the fundamentals and be prepared to demonstrate problem-solving skills during a Python interview. Good luck!
Frequently Asked Questions
What Are Some Common Pitfalls to Avoid When Working With Python?
When working with Python, avoid common pitfalls like improper indentation that can cause errors in your code.
Always remember to use clear variable names and stay organized to make debugging easier.
How Can You Improve the Performance of Your Python Code?
To improve the performance of your Python code, consider optimizing algorithms, minimizing unnecessary loops, using built-in functions efficiently, and employing data structures like sets and dictionaries.
Profile your code to identify bottlenecks and enhance speed.
What Are Some Best Practices for Debugging Python Applications?
When debugging Python applications, start by understanding the issue. Utilize print statements, logging, and debugging tools like pdb.
Break down the problem, test in small sections, and fix one issue at a time for effective debugging.
How Does Python Handle Memory Management?
Python handles memory management by using a private heap to store objects.
It has an automatic garbage collector to reclaim memory used by objects no longer in use.
You can also explicitly deallocate memory using the del
keyword.
Can You Explain the Global Interpreter Lock (Gil) in Python and Its Implications for Multi-Threading?
Sure!
The Global Interpreter Lock (GIL) in Python restricts the execution of multiple threads simultaneously.
This impacts multi-threading by allowing only one thread to execute at a time in a Python process, affecting performance in multi-core systems.
Conclusion
In conclusion, mastering these top 500 Python interview questions will greatly enhance your understanding of the language and prepare you for any job interview.
By solidifying your knowledge of Python basics, data structures, functions, modules, object-oriented programming, and advanced concepts, you’ll be well-equipped to tackle any coding challenge that comes your way.
Keep practising and honing your skills to become a Python expert in no time!